We will show you how to validate username and password in React JS in this simple blog post. In the concept, you will understand how to validate username and password in React JS. In this example of the blog, we will show you how to validate your username and password in React JS.
You’ve come to the right place if you want to see an example of React username and password validation!
Sometimes you may need to add username and password checks to React JS if your application has that field. This guide will show you step by step how to use React JS to validate a username that has at least 6 characters and no whitespace.
Step 1: Set Up Your React Project
- Open your CMD.
- Then create a new React JS project by running this command:
npx create-react-app username-password-validation
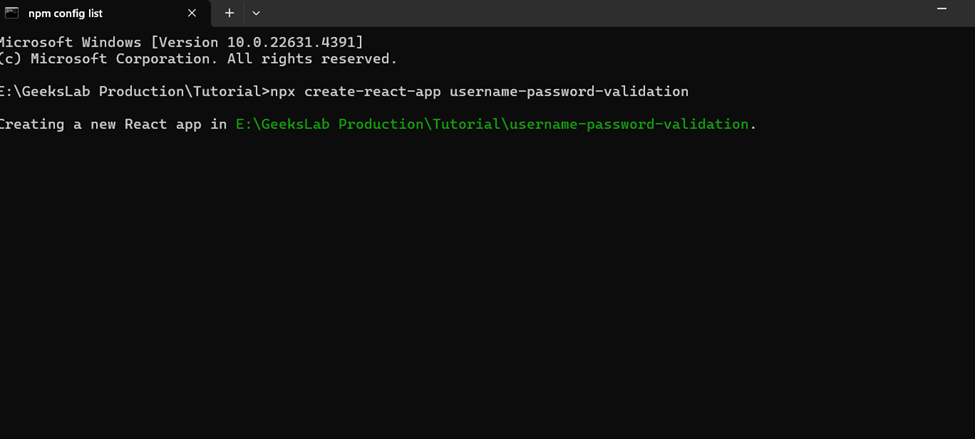
- Go to the project folder and run this command:
cd username-password-validation
- Open the project in your code editor. If you use VS code editor, run this command:
code .
Step 2: Create a Main Component
- Make a new file called LoginForm.js in the src folder.
- In LoginForm.js, add the following code to make a functional component called LoginForm:
import React, { useState } from 'react';
const LoginForm = () => {
return (
<div>
<h2>Login Form</h2>
</div>
);
};
export default LoginForm;
- In src/App.js, import LoginForm component, and you can see it in your browser:
import React from 'react';
import './App.css';
import LoginForm from './LoginForm';
function App() {
return (
<div className="App">
<LoginForm />
</div>
);
}
export default App;
- Start the development server by following this command:
npm start
Step 3: Add State for Username, Password, and Errors to track values
- In LoginForm.js component , set up the useState hook to track the username, password, and errors.
- Update the LoginForm.js component to include the state hooks:
import React, { useState } from 'react';
const LoginForm = () => {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const [errors, setErrors] = useState({});
return (
<div>
<h2>Login Form</h2>
</div>
);
};
export default LoginForm;
Step 4: Create Input Fields and Using useState hook for Error Messages
- You should add fields for entering the username and password, as well as error messages that will show up if the validation fails.
- Update the LoginForm component with this code:
import React, { useState } from 'react';
const LoginForm = () => {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const [errors, setErrors] = useState({});
return (
<div>
<h2>Login Form</h2>
<form>
<div>
<label>Username:</label>
<input
type="text"
value={username}
onChange={(e) => setUsername(e.target.value)}
/>
{errors.username && <p style={{ color: 'red' }}>{errors.username}</p>}
</div>
<div>
<label>Password:</label>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
{errors.password && <p style={{ color: 'red' }}>{errors.password}</p>}
</div>
<button type="submit">Login</button>
</form>
</div>
);
};
export default LoginForm;
Step 5: Add functions for validation
- Make functions that check the username and password against specific rules.
- Inside LoginForm.js component, add two functions: validateUsername and validatePassword. Follow the image to add this code.👇
const validateUsername = (username) => {
if (username.length < 3) {
return 'Username must be at least 3 characters long.';
}
return '';
};
const validatePassword = (password) => {
if (password.length < 8) {
return 'Password must be at least 8 characters long.';
}
if (!/[A-Z]/.test(password)) {
return 'Password must contain at least one uppercase letter.';
}
if (!/[a-z]/.test(password)) {
return 'Password must contain at least one lowercase letter.';
}
if (!/[0-9]/.test(password)) {
return 'Password must contain at least one number.';
}
if (!/[^A-Za-z0-9]/.test(password)) {
return 'Password must contain at least one special character.';
}
return '';
};
Step 6: Set up form submission and validation
- Then , add a handleSubmit arrow function to validate inputs and update errors. Follow the image to add this code. 👇
const handleSubmit = (event) => {
event.preventDefault();
const usernameError = validateUsername(username);
const passwordError = validatePassword(password);
if (usernameError || passwordError) {
setErrors({ username: usernameError, password: passwordError });
} else {
setErrors({});
alert('Login successful');
}
};
- Update the form. Go to the <form> tag and add handleSubmit function:
<form onSubmit={handleSubmit}>
Step 7: Full Component LoginForm.js Code here
You want to use the following final LoginForm.js file code. If you are very busy, copy and paste this code on your LoginForm.js file.
import React, { useState } from 'react';
const LoginForm = () => {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const [errors, setErrors] = useState({});
const validateUsername = (username) => {
if (username.length < 3) {
return 'Username must be at least 3 characters long.';
}
return '';
};
const validatePassword = (password) => {
if (password.length < 8) {
return 'Password must be at least 8 characters long.';
}
if (!/[A-Z]/.test(password)) {
return 'Password must contain at least one uppercase letter.';
}
if (!/[a-z]/.test(password)) {
return 'Password must contain at least one lowercase letter.';
}
if (!/[0-9]/.test(password)) {
return 'Password must contain at least one number.';
}
if (!/[^A-Za-z0-9]/.test(password)) {
return 'Password must contain at least one special character.';
}
return '';
};
const handleSubmit = (event) => {
event.preventDefault();
const usernameError = validateUsername(username);
const passwordError = validatePassword(password);
if (usernameError || passwordError) {
setErrors({ username: usernameError, password: passwordError });
} else {
setErrors({});
alert('Login successful');
}
};
return (
<div>
<h2>Login Form</h2>
<form onSubmit={handleSubmit}>
<div>
<label>Username:</label>
<input
type="text"
value={username}
onChange={(e) => setUsername(e.target.value)}
/>
{errors.username && <p style={{ color: 'red' }}>{errors.username}</p>}
</div>
<div>
<label>Password:</label>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
{errors.password && <p style={{ color: 'red' }}>{errors.password}</p>}
</div>
<button type="submit">Login</button>
</form>
</div>
);
};
export default LoginForm;
Note: Make sure the validation works perfectly if you submit a blank form. You will see like this. 👇