It is very important that password fields on web forms are safe and easy to use. One way to improve security is to use “password” and “confirm password” validation.In this guide, we will show you how to make a React component that checks input fields.
Step 1: Install and Set Up Your React App
First, open CMD, run this command, and create a new React app.
npx create-react-app password-validation-app
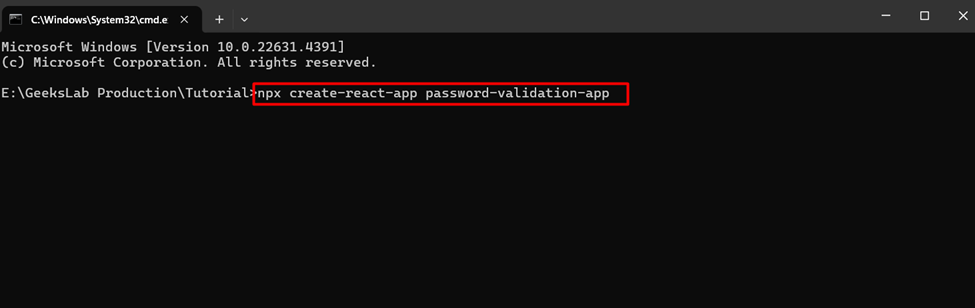
Step 2: Go to the project folder :
cd password-validation-app
Step 3: Set up the Form Component :
Go to the src folder. In the src folder, create a new file named PasswordForm.js.
Step 4: Paste this code :
I breakdown this code before pasting: 👇
- Importing necessary libraries and styles: Setting up React, useState, and a custom CSS file by importing the necessary libraries and styles.
- Primary function: Sets up the PasswordForm function component.
- State for input values and errors: Initializes input and errors as state variables for managing form data and validation error messages.
- Handle changes in input fields: Defines handleChange, which updates input state whenever a user types in any form field.
- Handle form submission: Defines handleSubmit to prevent page reload, validate the form, and reset fields on successful validation.
- Validate form inputs: Defines validate to check:
- Name field (required),
- Email format and requirement,
- Password requirement and match with confirm password.
- Name validation – required field: Ensures the name field is filled in.
- Email validation – required and must match email format: Checks for a valid email address format if an email is provided.
- Password validation – required field: Confirms the password field is not empty.
- Confirm Password validation – required and must match password: Ensures that the confirm password field matches the password field.
- Set error messages if any: Updates errors state with validation errors.
- Render form with inputs and error messages: Renders the form with each input field and displays validation error messages, plus a submit button.
Then paste this code in PasswordForm.js file.👇
import React, { useState } from 'react';
import './PasswordForm.css';
function PasswordForm() {
const [input, setInput] = useState({ name: '', email: '', password: '', confirmPassword: '' });
const [errors, setErrors] = useState({});
const handleChange = (event) => {
const { name, value } = event.target;
setInput({ ...input, [name]: value });
};
const handleSubmit = (event) => {
event.preventDefault();
if (validate()) {
alert('Form submitted successfully!');
setInput({ name: '', email: '', password: '', confirmPassword: '' });
}
};
const validate = () => {
const newErrors = {};
let isValid = true;
if (!input.name) {
isValid = false;
newErrors.name = 'Please enter your name.';
}
if (!input.email) {
isValid = false;
newErrors.email = 'Please enter your email address.';
} else {
const emailPattern = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
if (!emailPattern.test(input.email)) {
isValid = false;
newErrors.email = 'Please enter a valid email address.';
}
}
if (!input.password) {
isValid = false;
newErrors.password = 'Please enter your password.';
}
if (!input.confirmPassword) {
isValid = false;
newErrors.confirmPassword = 'Please confirm your password.';
} else if (input.password !== input.confirmPassword) {
isValid = false;
newErrors.password = "Passwords don't match.";
}
setErrors(newErrors);
return isValid;
};
return (
<div className="form-container">
<h2>Password and Confirm Password Validation in React JS</h2>
<form onSubmit={handleSubmit}>
<div className="form-group">
<label>Name:
<input
type="text"
name="name"
value={input.name}
onChange={handleChange}
placeholder="Enter name"
/>
</label>
<div className="error">{errors.name}</div>
</div>
<div className="form-group">
<label>Email:
<input
type="email"
name="email"
value={input.email}
onChange={handleChange}
placeholder="Enter email"
/>
</label>
<div className="error">{errors.email}</div>
</div>
<div className="form-group">
<label>Password:
<input
type="password"
name="password"
value={input.password}
onChange={handleChange}
placeholder="Enter password"
/>
</label>
<div className="error">{errors.password}</div>
</div>
<div className="form-group">
<label>Confirm Password:
<input
type="password"
name="confirmPassword"
value={input.confirmPassword}
onChange={handleChange}
placeholder="Confirm password"
/>
</label>
<div className="error">{errors.confirmPassword}</div>
</div>
<button type="submit" className="submit-btn">Submit</button>
</form>
</div>
);
}
export default PasswordForm;
Step 5: Add Custom CSS
Create a CSS file to style your form. This file’s name is PasswordForm.css in the src folder for custom styling. Add the following code and paste it on this file:
/* PasswordForm.css */
.form-container {
max-width: 400px;
margin: 50px auto;
padding: 20px;
border-radius: 8px;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.1);
background-color: #f9f9f9;
}
h2 {
text-align: center;
color: #333;
}
.form-group {
margin-bottom: 15px;
}
label {
font-weight: bold;
display: block;
margin-bottom: 5px;
color: #333;
}
input[type="text"],
input[type="email"],
input[type="password"] {
width: 100%;
padding: 8px;
border: 1px solid #ccc;
border-radius: 4px;
font-size: 16px;
}
input[type="text"]:focus,
input[type="email"]:focus,
input[type="password"]:focus {
outline: none;
border-color: #007bff;
}
.error {
color: #d9534f;
font-size: 14px;
margin-top: 5px;
}
.submit-btn {
width: 100%;
padding: 10px;
border: none;
background-color: #007bff;
color: #fff;
font-size: 16px;
border-radius: 4px;
cursor: pointer;
transition: background-color 0.3s;
}
.submit-btn:hover {
background-color: #0056b3;
}
Step 6: Import and Use the Component on your parent Component
In this case, my parent component is App.js. So, I import the PasswordForm component in App.js component.
import React from 'react';
import PasswordForm from './PasswordForm';
function App() {
return (
<div className="App">
<PasswordForm />
</div>
);
}
export default App;
Step 5: Run Application
Finally, start the application by running this command :
npm start
Finally, you show like this form. 👇
Conclusion
This guide shows a full example of setting up password and confirm password validation in React JS and using custom CSS . Actually custom CSS use for the styling.