If you are new to web development and want to create a fully dynamic website, Then PHP might be an excellent programming language for you. PHP (Hypertext Preprocessor) is a server-side scripting language that is specially designed for basic to high end web development. In today’s post we will learn about the basics of PHP, how PHP loops, variables and functions work. We will see how to create a landing page by coding with PHP. Let’s start learning PHP.
What is PHP?
Php is a widely used open source scripting language. It is possible to create any type of dynamic website using PHP embedded with HTML. Only the static content of a website can be created with HTML. There any type of interactive design can be created by adding PHP with HTML. PHP can be used to do a lot more, including handling forms, connecting to a website’s database, session control and others.
Reasons why PHP is so popular:
- Open Source: PHP is free to use and has a large community that offers support.
- Easy to Learn: PHP’s syntax is relatively easy for beginners to grasp.
- Cross-Platform: PHP runs on various platforms, including Windows, macOS, and Linux.
- Compatible with Databases: PHP works seamlessly with MySQL and other databases.
How PHP Works
PHP is executed on the server, which means the code you write is processed by the server and only the resulting HTML is sent to the browser. This ensures that the user never sees the PHP code itself, just the output.
Here’s a basic flow:
- A user requests a web page (for example, index.php).
- The server identifies that the file contains PHP code and processes it.
- The PHP code is executed, interacting with the database or performing other tasks.
- The server returns the resulting HTML to the user’s browser.
Setting Up PHP
Before you can start writing PHP code, you need a server where PHP can be processed. You can use it in two ways:
1. Use a Local Server: Tools like XAMPP or WAMP allow you to run a server on your computer for development purposes.
- XAMPP: A package that includes Apache (server), MySQL (database), and PHP. Download it from XAMPP.
- WAMP: Similar to XAMPP but for Windows. Download it from WAMP.
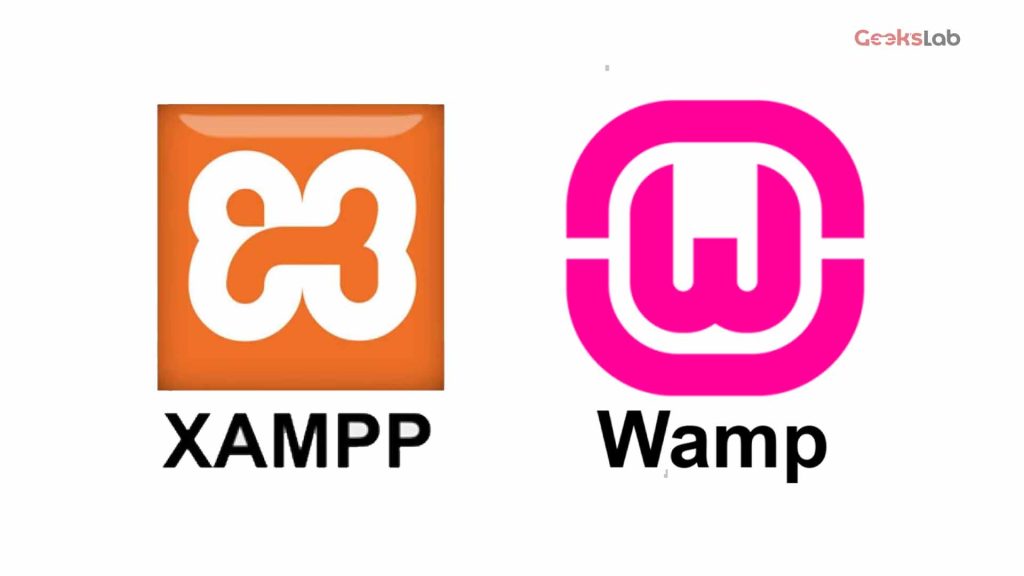
2. Use a Web Hosting Service: If you have web hosting, most providers offer PHP support out of the box.
Your First PHP Script
Let’s dive into the basics of PHP by writing a simple script. The following example demonstrates how to embed PHP code within an HTML document.
<!DOCTYPE html>
<html lang="en">
<head>
<title>My First PHP Page</title>
</head>
<body>
<h1>Hello World!</h1>
<?php
echo "This is my first PHP script!";
?>
</body>
</html>
Explanation:
- The code above includes HTML structure and a small PHP block inside the <body> tag.
- The <?php … ?> tags enclose the PHP code.
- The echo statement outputs the text to the browser.
PHP Variables
Variables in PHP start with a dollar sign ($) and can store different types of data, like strings, numbers, or arrays. Let’s look at an example:
<?php
$name = "John";
$age = 25;
echo "My name is $name and I am $age years old.";
?>
Comments in PHP
PHP supports single-line and multi-line comments. These are useful for adding notes or explaining parts of your code.
// This is single line comment
/*
This is a
Multi-line comment
*/
Conditional Statements
Like other programming languages, PHP includes conditional statements such as if, else, and elseif.
<?php
$score = 75;
if ($score >= 80) {
echo "You passed with an A!";
}
elseif ($score >= 60) {
echo "You passed with a B!";
}
else {
echo "You failed";
}
?>
Loops in PHP
PHP supports various loops (for, while, do-while) to repeat a block of code multiple times.
Here’s an example using a for loop:
<?php
for ($i = 1; $i <= 5; $i++) {
echo "This is loop iteration $i <br>";
}
?>
Working with Forms
PHP can process user input from HTML forms. Here’s a simple example of handling a form submission:
In this example, the form sends a POST request to process. php, where PHP processes the input.
<form action="process.php" method="POST">
Name: <input type="text" name="name">
<input type="submit" value="Submit">
</form>
PHP (process.php)
<?php
$name = $_POST['name']; // Get the submitted value
echo "Hello, $name!";
?>
PHP is a very powerful server side scripting language. By learning PHP, you can develop interactive websites using the variables, conditional statements, loops, and form handling.
We will discuss every function in detail in our next post.