npm install -D tailwindcss postcss autoprefixer
An easy-to-follow guide on how to add Tailwind CSS to React project. When building React applications, Tailwind CSS makes for a great utility-first classes for fast UI development. A step-by-step guide on how to attach Tailwind CSS with your React project.
Step 1: Create a New Vite + React Project
Then create a new Vite project with the React framework. Open terminal, then commend this:
npm create vite@latest my-tailwind-app -- --template react
cd my-tailwind-app
npm install
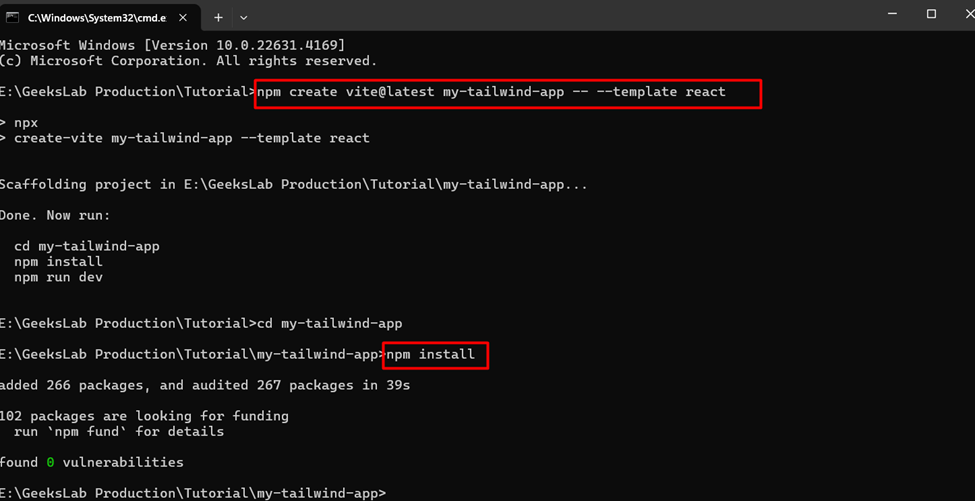
This will create a new Vite project and choose the React framework. Then your folder should look like this.
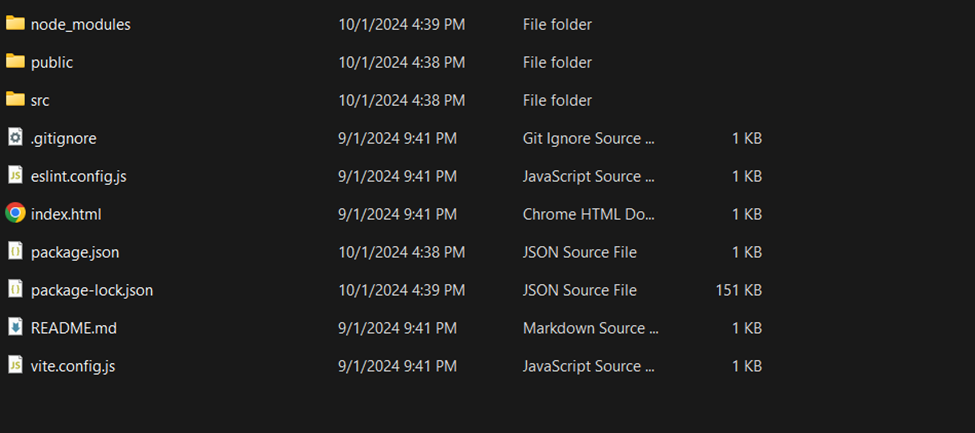
Step 2 : Install Tailwind CSS
In your project folder, install Tailwind CSS along with its dependencies:
npm install -D tailwindcss postcss autoprefixer
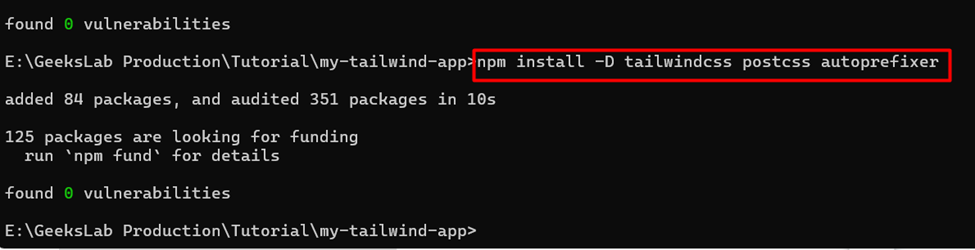
This will create your tailwind.config.js and postcss.config.js files.
npx tailwindcss init -p
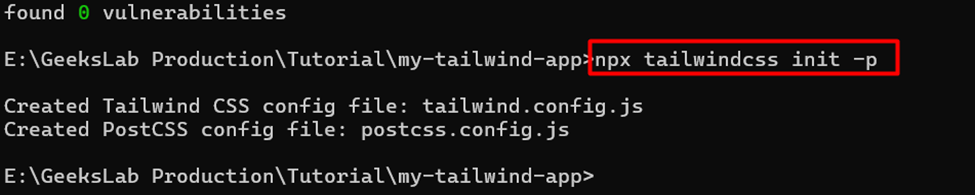
Step 3 : Configure Tailwind CSS
Next, you have to add Tailwindcss to purge unused styles for production and scan all your files looking for tailwind classes.
In the project folder, open tailwind.config.js file, replace the content configuration with:
module.exports = {
content: [
'./index.html',
'./src/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {},
},
plugins: [],
}
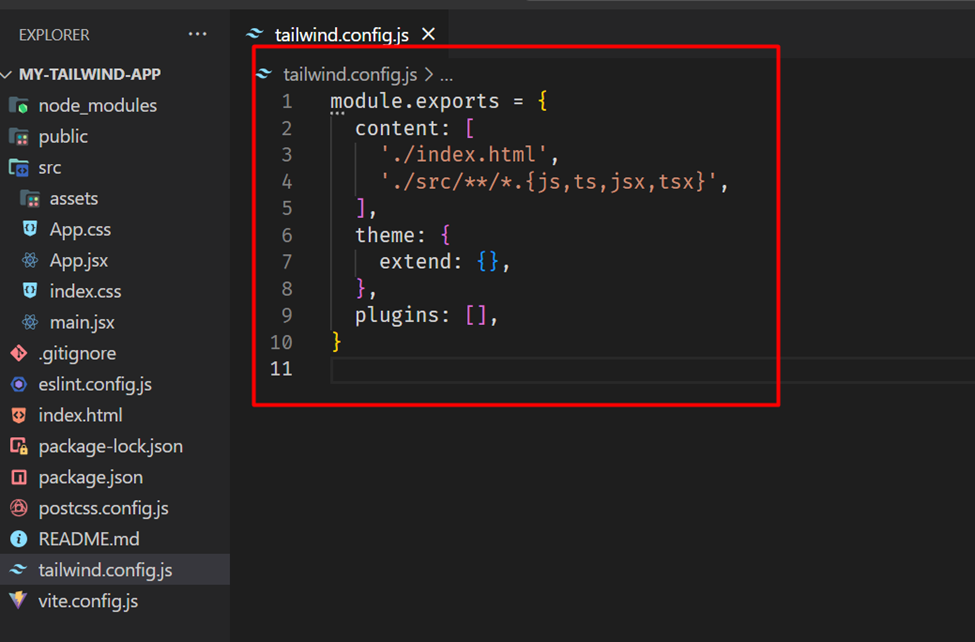
🌟This makes sure that Tailwind looks at your main index file. Like main.jsx and app.jsx. Must be all JavaScript/TypeScript files inside the src folder.
4. Set Up Tailwind in Your Main CSS File
In your project’s src folder, create or open the index.css file. And add the following lines to import Tailwind’s base, components, and utility styles:
@tailwind base;
@tailwind components;
@tailwind utilities;
🌟That will include Tailwind styles in your project.
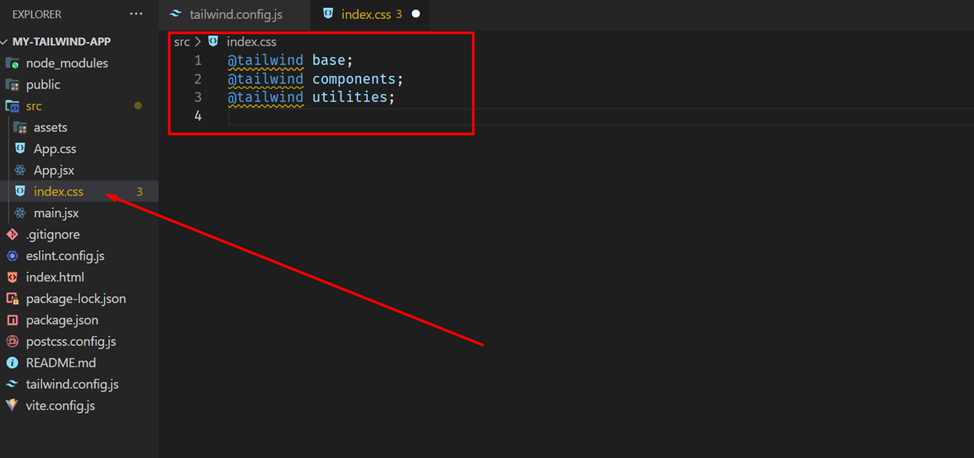
Step 5: Start the Development Server
Now, you can start using Tailwind CSS in your React app with Vite. Run the development server with the following command:
npm run dev
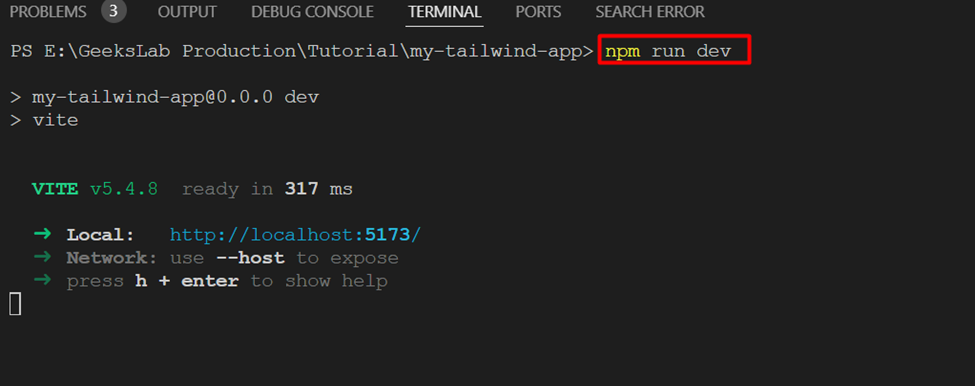
Now you can use Tailwind’s utility classes in your React components because the fast development server for Vite will be up and running.
Step 6: Example Usage of Tailwind CSS in React Your React Project
In this below is an example React component using Tailwind CSS classes:
import './App.css'
function App() {
return (
<div className="min-h-screen flex items-center justify-center bg-gray-100">
<div className="bg-white p-10 rounded-lg shadow-md">
<h1 className="text-2xl font-bold text-gray-800">
Tailwind with Vite and React!
</h1>
<p className="text-gray-600 mt-4">
This is a simple setup to use Tailwind CSS in a Vite project with React Project.
</p>
<button className="mt-6 bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-600">
Get Started
</button>
</div>
</div>
);
}
export default App
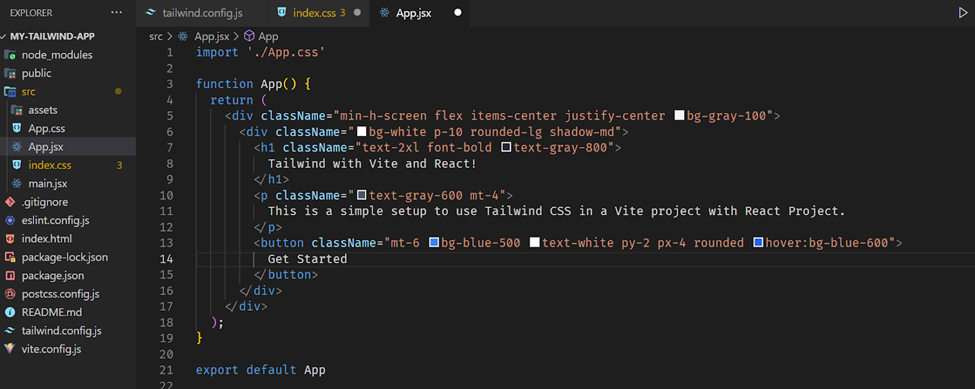
7. Build for Production
When you’re ready to deploy your project, run the following command to build your project for production:
npm run build
This is to production build your project and remove the unused CSS that was tree shaken in order to have the smallest final bundle.
Conclusion : Between Vite and Tailwind CSS + React, you have a performant AND productive development setup. Combining Vite’s super-fast build times with Tailwind’s utility-first approach to styling, you can use it to spin up web applications in no time.
Go ahead and try out Tailwind classes in your React app now that everything is set up!