Integrating external API data into a WordPress site can be done in multiple ways, depending on your needs.
Following the below steps, how you can fetch and display data from an external API in WordPress. We will do this in a live server without any wordpress plugin but you can do this in localhost also.
Step 1: WordPress functions:
You can use wp_remote_get() or wp_remote_post() to fetch data from an API and display it on your WordPress site.
We will use https://jsonplaceholder.typicode.com/users this link for users data and our shortcode will be ‘external_data’.
Step 2: Use shortcode:
Create a new page and put the shortcode in the page as shown in the image below. You can use the shortcode in your existing page or where you want to show the data.
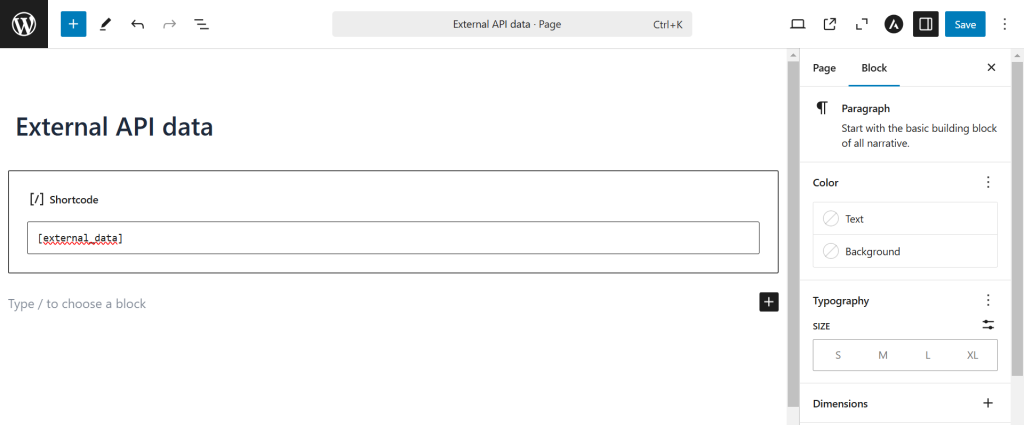
Step 3: Edit functions.php file:
From your wordpress dashboard, go to Appearance> Theme file editor and find functions.php file as in the below image.
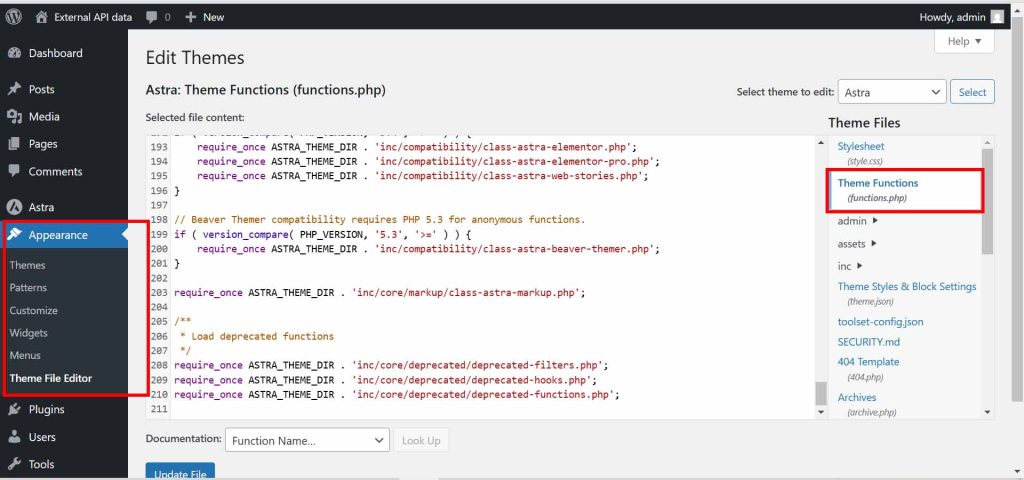
Now, at the end of the code, paste the below code to functions.php file and update.
/**
* External API data in wordpress
*/
defined('ABSPATH') || die('Unauthorized Access');
<!-- Action when user logs into admin panel -->
add_shortcode('external_data', 'callback_function_name');
function callback_function_name( $atts ) {
if ( is_admin() ) {
return '<p>This is where the shortcode [external_data] will show up.</p>';
}
$defaults = [
'title' => 'Users title'
];
$atts = shortcode_atts(
$defaults,
$atts,
'external_data'
);
$url = 'https://jsonplaceholder.typicode.com/users';
$arguments = array(
'method' => 'GET'
);
$response = wp_remote_get($url, $arguments);
if (is_wp_error($response) ) {
$error_message = $response->get_error_message();
return "Something went wrong: $error_message";
}
$results = json_decode( wp_remote_retrieve_body($response) );
$html = '<h2>' . $atts['title'] . '</h2>
<table>
<tr>
<td>id</td>
<td>Name</td>
<td>Username</td>
<td>Email</td>
<td>Address</td>
</tr>';
foreach( $results as $result ) {
$html .= '<tr>';
$html .= '<td>' . $result->id . '</td>';
$html .= '<td>' . $result->name . '</td>';
$html .= '<td>' . $result->username . '</td>';
$html .= '<td>' . $result->email . '</td>';
$html .= '<td>' . $result->address->street . ', ' . $result->address->suite . ', ' . $result->address->city . ', ' . $result->address->zipcode . '</td>';
$html .= '</tr>';
}
$html .= '</table>';
return $html;
}
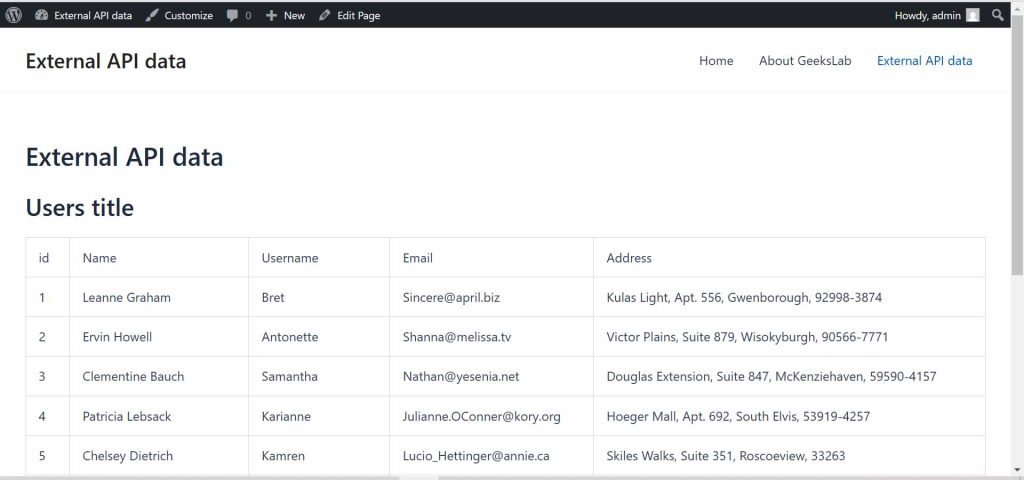
Go to that page where you put the shortcode and reload the page. Your data should be on the page. By using this technique, you can create more API in your website.