Converting a static HTML/CSS site into a React app is about transforming your existing HTML, CSS, and JavaScript to a React components. Here’s a step-by-step tutorial to achieve this.
Steps to Convert a Static HTML/CSS Site to a React App
Step 1: At First, Set Up a New React Project
First, you need to set up a React environment using create-react-app or Vite . I personally prefer to use vite for your speedy production. This command or app gives you a ready-to-use environment for building your react app.
Set Up a New React Project
- First, you need to set up a React environment using npm create vite@latest.
- Then , enter your project name for your project.
- Then , Select React
- Then, Select JavaScript
- Then run this command: npm install
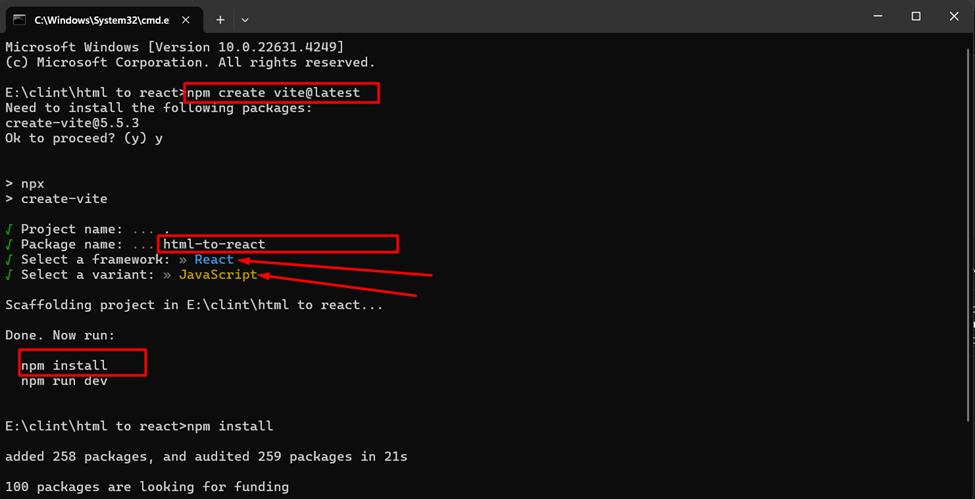
Step 2: Organize and split your static HTML file
- Like this is your HTML Code. 👇
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Static Site</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
<nav>
<a href="#">Home</a>
<a href="#">About</a>
<a href="#">Contact</a>
</nav>
</header>
<section id="main-content">
<p>This is the main content of the site.</p>
</section>
<footer>
<p>© 2024 My Website</p>
</footer>
</body>
</html>
- Then break down HTML into React components for reusable parts. For example, you might have a Header, Footer, and MainContent component.
React Component Structure
- Create a Header.jsx or js file for the header.
- Create a Footer.jsx or js file for the footer.
- Create a MainContent.jsx or js file for the main content.
N/B: Please all components file names must be capital letters.
Step 3: Create Componants:
Create Header Componants: Now you can create Header components for your project.
Header Component Structure (src/components/Header.jsx):
import React from 'react';
const Header = () => {
return (
<header>
<h1>Welcome to My Website</h1>
<nav>
<a href="#">Home</a>
<a href="#">About</a>
<a href="#">Contact</a>
</nav>
</header>
);
};
export default Header;

Create Footer Componants: Now you can create Footer components for your project.
Footer Component Structure (src/components/Footer.jsx):
import React from 'react';
const Footer = () => {
return (
<footer>
<p>© 2024 My Website</p>
</footer>
);
};
export default Footer;
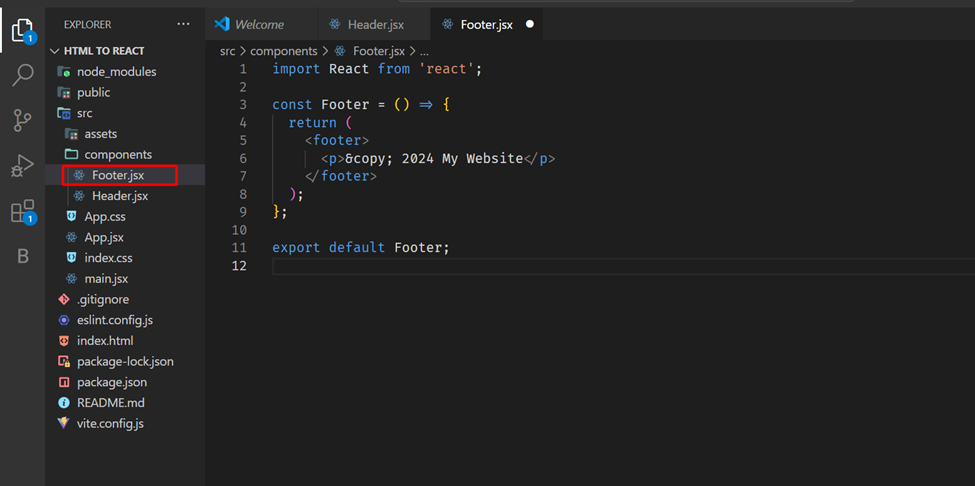
Create MainContent Componants: Now you can create MainContent components for your project.
MainContent Component Structure (src/components/MainContent.jsx):
import React from 'react';
const MainContent = () => {
return (
<section id="main-content">
<p>This is the main content of the site.</p>
</section>
);
};
export default MainContent;
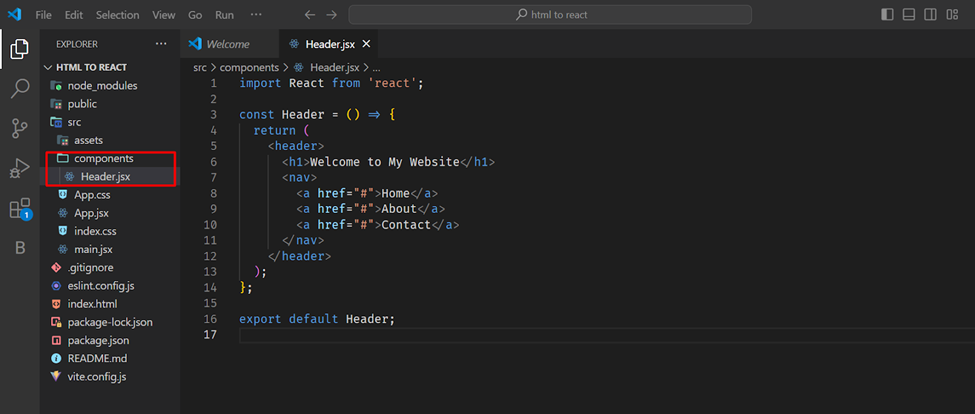
Create Footer Componants: Now you can create Footer components for your project.
Footer Component Structure (src/components/Footer.jsx):
import React from 'react';
const Footer = () => {
return (
<footer>
<p>© 2024 My Website</p>
</footer>
);
};
export default Footer;
); }; export default Footer;
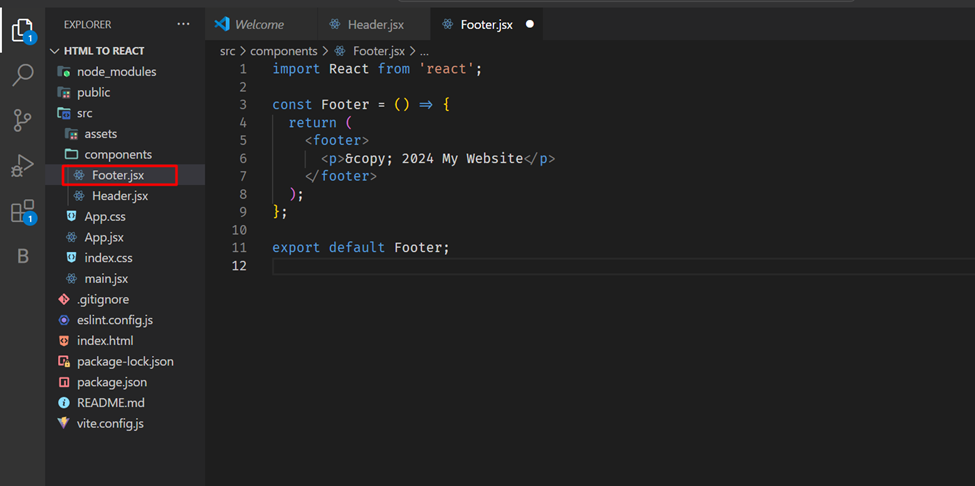
Create MainContent Componants: Now you can create MainContent components for your project.
MainContent Component Structure (src/components/MainContent.jsx):
import React from 'react';
const MainContent = () => {
return (
<section id="main-content">
<p>This is the main content of the site.</p>
</section>
);
};
export default MainContent;
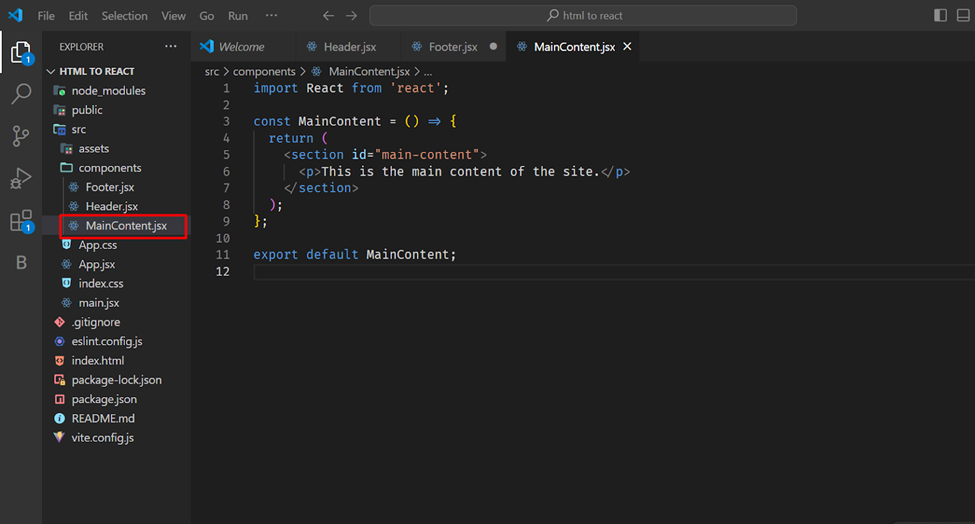
Step 4: Create Your App Component
Now that you have your components, you can adjust them in your App.jsx file.
import React from 'react';
import Header from './components/Header';
import MainContent from './components/MainContent';
import Footer from './components/Footer';
import './styles/style.css'; // Import your CSS file here
function App() {
return (
<div className="App">
<Header />
<MainContent />
<Footer />
</div>
);
}
export default App;
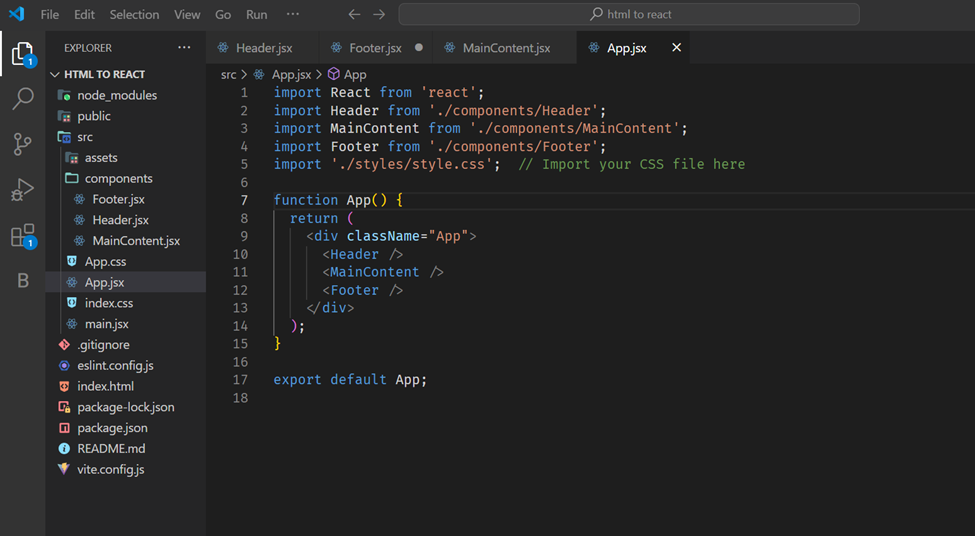
Step 5: Add Style File
Create style.css file in the src/styles folder and import it into your App.jsx file:
import './styles/style.css';
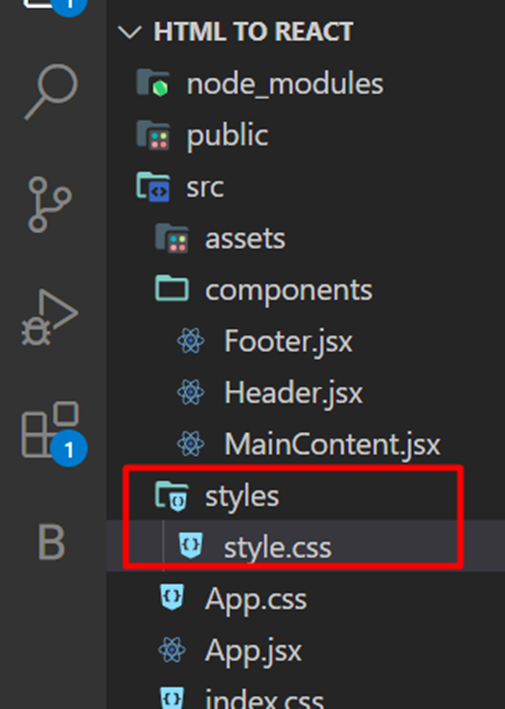
Step 6: Handling Images and All Assets
For images, you can refer to using the public folder or import them into components:
- Place your images in the public folder and reference them like so in your components:
- <img src=”/path/to/image.jpg” alt=”Description” />
- Alternatively, you can import images into React components like this:
import Image from './assets/my-image.jpg';
const Component = () => {
return <img src={Image} alt="Description" />;
};
Step 7: Deploy Or Build Your React App
You can use Vercel, Netlify, or traditional hosting services to put your React app online once it is ready. To build your app for production , apply this command:
npm run build
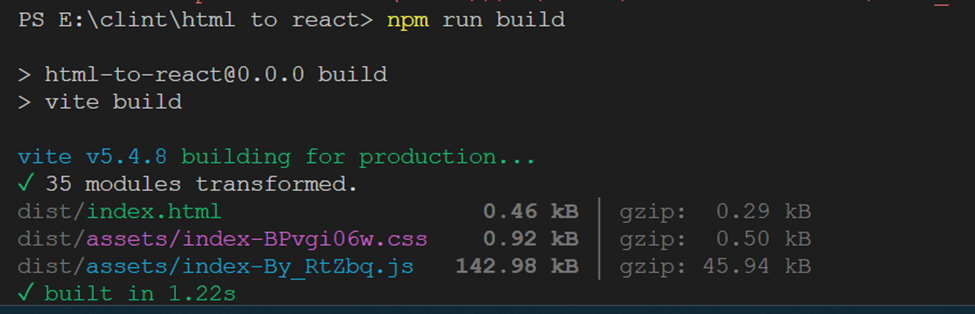
This command will generate a build folder, which contains the production-ready static files.
Conclusion: To turn a static HTML/CSS site into a React app, you need to organize your assets, break the site down into reusable parts, and use React’s state and props as needed. React not only makes it easier to organize code, but it also makes it easier to make changes in the future.